2022. 8. 30. 10:55ㆍiOS/Learning
[Xcode] 실행 -> Create a new Xcode project
or
[Xcode] 상단바 -> File -> New -> Project
-> initialize 완료!
프로젝트 구성
화면 왼쪽 네비게이터 영역
- AppDelegate(.swift): App Life Cycle 관리, 앱 실행 ~ 종료/백그라운드 실행시 작업 관리
- SceneDelegate(.swift): UI Life Cycle 관리
- ViewController(.swift): 화면(뷰)에서 처리하는 작업 관리 -> 여기서 코딩할거임
- Main(.storyboard): 스토리보드: 화면에 보이는 내용, 뷰와 뷰의 연결 관계 등을 그림으로 표현, 시각적인 이해에 도움됨
- Assets(.xcassets): 앱 아이콘 저장소 -> 여기서 앱 아이콘 설정해야 함
- LaunchScreen(.storyboard): 앱 실행 시작시 나타나는 스플래시 화면의 스토리보드
- Info(.plist): 앱 실행 정보 파일
Lamp Aleart Example
전구 껐다 켰다하는 데 Alert(경고 메시지) 표시하는 앱 만들거임
1. 아래이미지 파일 다운
lamp image files
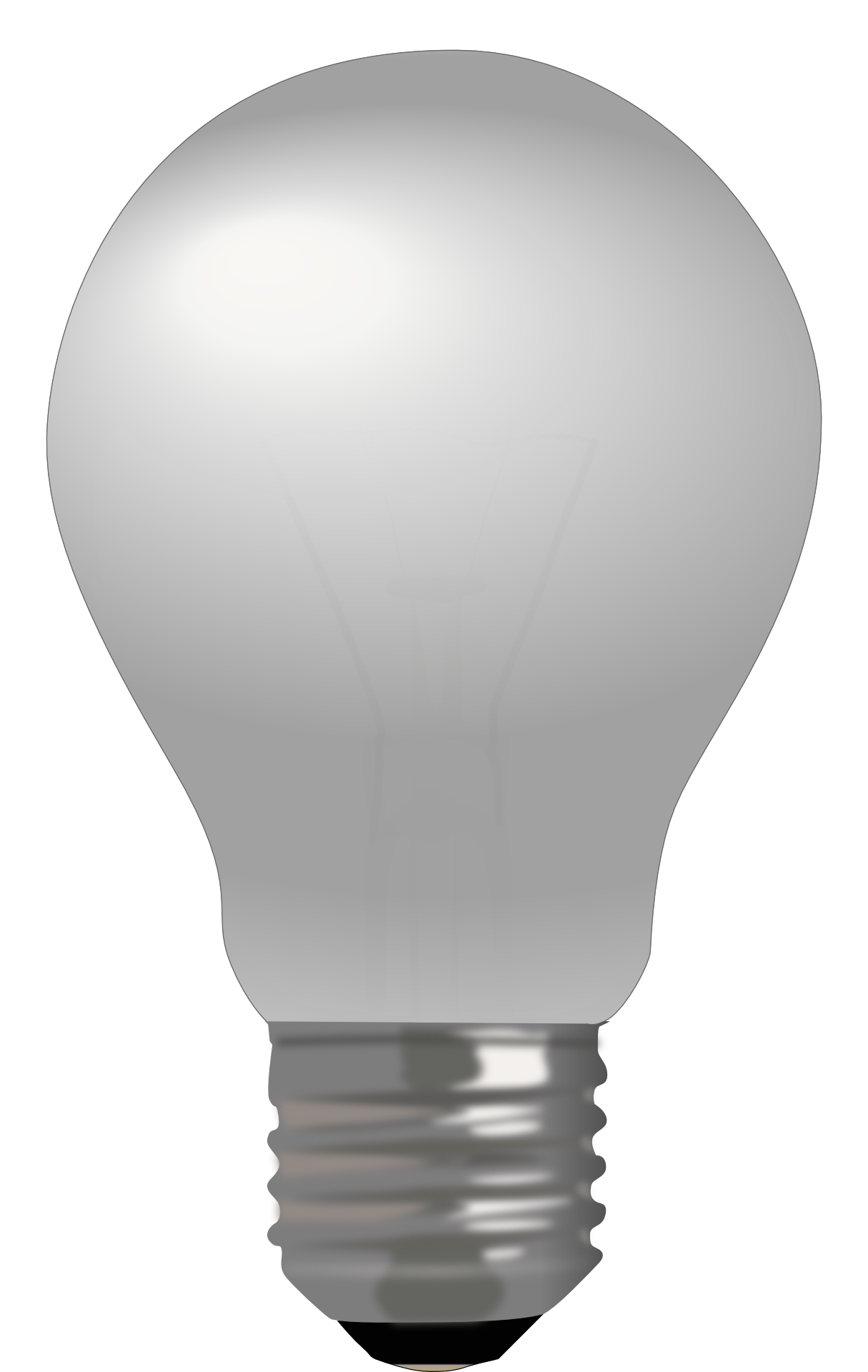
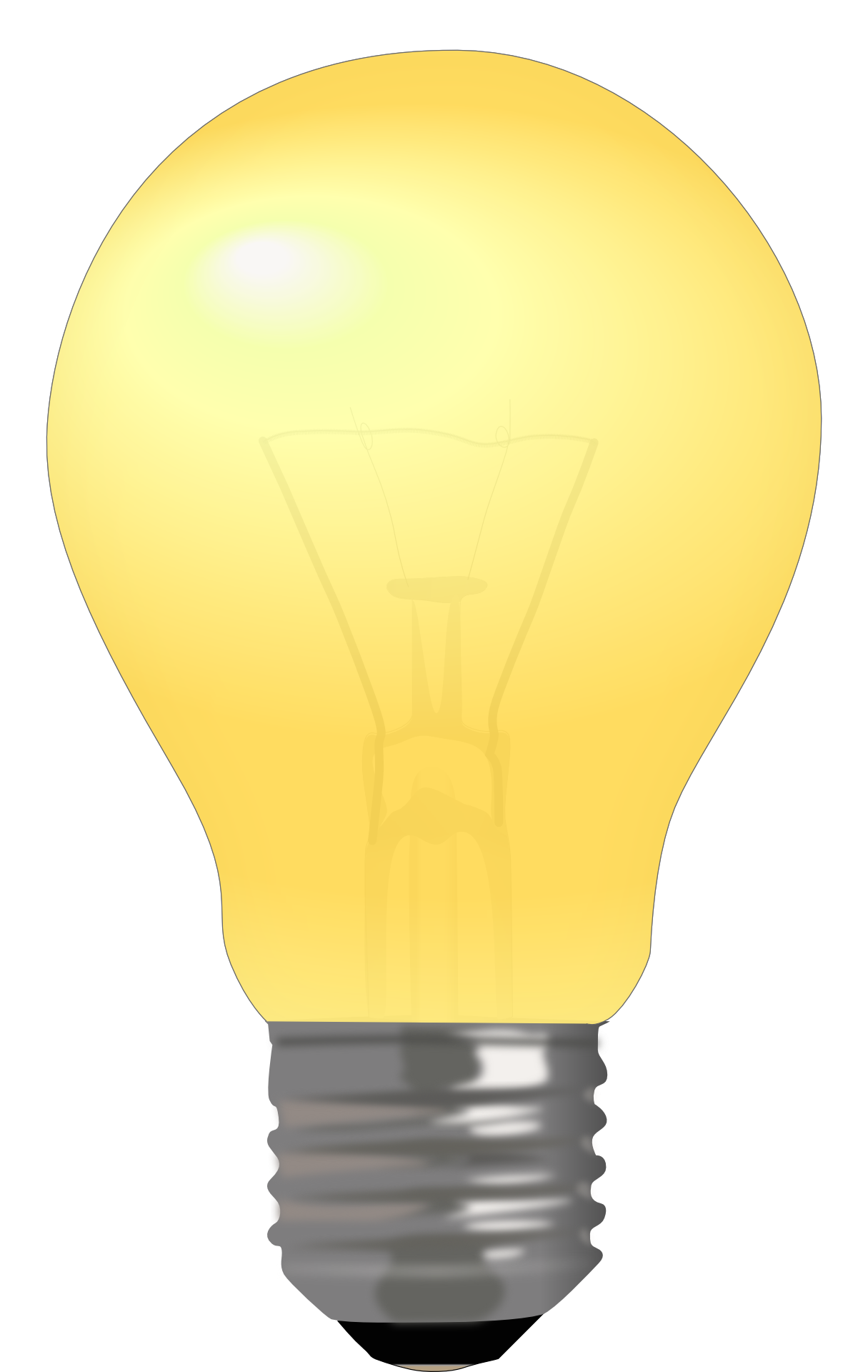
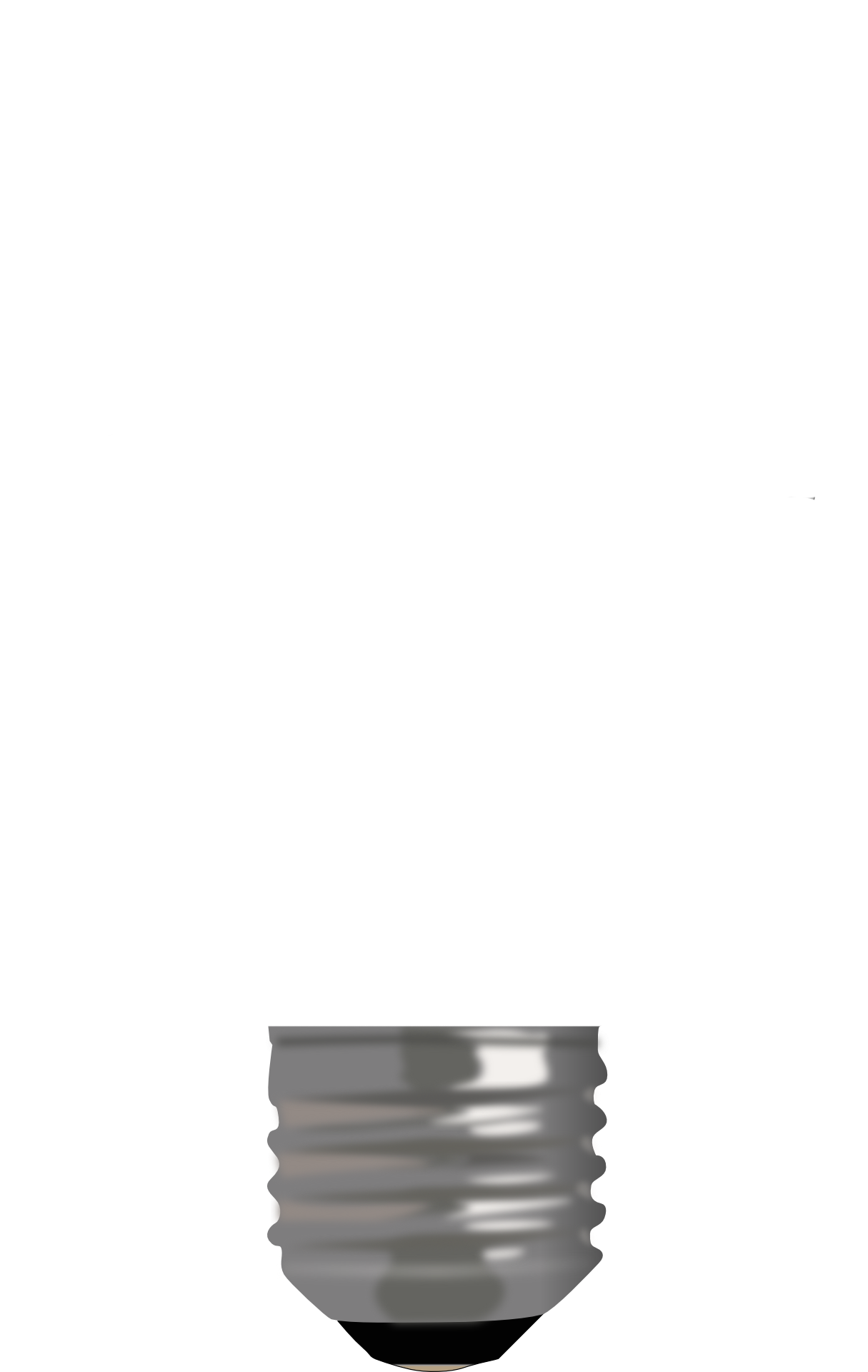
2. Finder에서 다운받은 이미지를 선택하여 Navigator 영역으로 Drag & Drop
3. Navigator 영역의 Main 스토리보드 -> 상단 [+] Library 버튼 클릭
3. button 도 마찬가지로 3개가져와서 ON, OFF, REMOVE로 Title 바꾸삼. 버튼은 스토리보드로 가져온다음에 더블클릭하면 Title text 바꿀 수 있음
4. Navigator 영역의 Main 스토리보드 -> 상단에 Adjust Editor Option 아이콘 -> Assistant
5. control 키 누른채로 스토리보드에서 각 컴포넌트를 클릭하여 Assistant 코드 영역으로 Drag & Drop하면 아웃렛 변수, 액션 함수가 추가됨
- Outlet Variable: 스토리 보드에 추가한 객체를 선택하고 내용을 변경하거나 특정 동작을 수행할 수 있도록 해당 객체에 접근하는 변수
- Action Function: 컴포넌트에 대한 액션을 수행했을때 실행할 동작을 정의하는 함수
ImageView 는 Outlet으로 추가
Connection | Outlet |
Name | lampImg |
Type | UIImageVeiw |
Button은 Action으로 추가
Connection | Action |
Name | lampOn |
Type | UIButton |
6. source code
// ViewController.swift
import UIKit
class ViewController: UIViewController {
@IBOutlet var lampImg: UIImageView!
let imgOn = UIImage(named: "lamp-on.png")
let imgOff = UIImage(named: "lamp-off.png")
let imgRemove = UIImage(named: "lamp-remove.png")
var isLampOn = true
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
lampImg.image = imgOn
}
@IBAction func lampOn(_ sender: UIButton) {
if isLampOn {
let lampOnAlert = UIAlertController(title: "Warnning", message: "It's already On.", preferredStyle: UIAlertController.Style.alert)
let onAction = UIAlertAction(title: "OK", style: UIAlertAction.Style.default, handler: nil)
lampOnAlert.addAction(onAction)
present(lampOnAlert, animated: true, completion: nil)
} else {
lampImg.image = imgOn
isLampOn = true
}
}
@IBAction func lampOff(_ sender: UIButton) {
if isLampOn {
let lampOffAlert = UIAlertController(title: "Turn Off", message: "Do you want to turn off the lamp?", preferredStyle: UIAlertController.Style.alert)
let offAction = UIAlertAction(title: "Yes", style: UIAlertAction.Style.default, handler: {ACTION in self.lampImg.image = self.imgOff
self.isLampOn = false
})
let cancelAction = UIAlertAction(title: "No", style: UIAlertAction.Style.default, handler: nil)
lampOffAlert.addAction(offAction)
lampOffAlert.addAction(cancelAction)
present(lampOffAlert, animated: true, completion: nil)
}
}
@IBAction func removelamp(_ sender: UIButton) {
let lampRemoveAlert = UIAlertController(title: "Remove Lamp", message: "Do you want to remove the lamp?", preferredStyle: UIAlertController.Style.alert)
let offAction = UIAlertAction(title: "No, Turn it off.", style: UIAlertAction.Style.default, handler: {
ACTION in self.lampImg.image = self.imgOff
self.isLampOn = false
})
let onAction = UIAlertAction(title: "No, Turn it on.", style: UIAlertAction.Style.default, handler: {
ACTION in self.lampImg.image = self.imgOn
self.isLampOn = true
})
let removeAction = UIAlertAction(title: "Yes.", style: UIAlertAction.Style.destructive, handler: {
ACTION in self.lampImg.image = self.imgRemove
self.isLampOn = false
})
lampRemoveAlert.addAction(offAction)
lampRemoveAlert.addAction(onAction)
lampRemoveAlert.addAction(removeAction)
present(lampRemoveAlert, animated: true, completion: nil)
}
}
- Closure(Anonymous Functions) : 효율적 코드 작성을 위해 함수 선언 없이 바로 몸체를 만들어 사용하는 일회용 함수
(lampOff 함수의 offAction의 handler)
{
ACTION in self.lampImg.image = self.imgOff
self.isLampOn = false
}
예시)
func competeWork(finished: Bool) -> () {
print("complete: \(finished)")
}
// 함수 이름 생략 ⬇️
/**
* (parameter) -> returnType in ... */
{
(finished: Bool) -> () in print("complete: \(finished)")
}
// 리턴타입, 파라미터 생략(컴파일러가 타입을 미리 알고 있는 경우), 괄호 생략 ⬇️
/**
* parameter in ... */
{
finished in print("complete: \(finished)")
}
7. 실행 결과
Reference
Do it! 스위프트로 아이폰 앱 만들기 입문 개정 4판, 이지스 퍼블리싱, 송호정, 이범근